
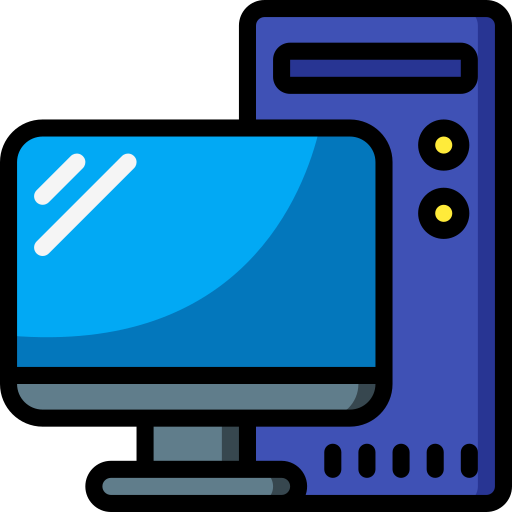
14·
11 months agoHello, sponsorblockcast creator here! Happy to finally see a Go implementation, great work! I ended up writing a short blog post summarising the history of the SponsorBlock clients for Chromecasts.
Best of luck in your continued development!
Part 1 felt fairly pretty simple in Haskell:
Part 2 was more of a struggle, though I’m pretty happy with how it turned out. I ended up using
concatMap inits . tails
to generate all substrings, in order of appearance soone3m
becomes["","o","on","one","one3","one3m","","n","ne","ne3","ne3m","","e","e3","e3m","","3","3m","","m",""]
. I then wrote a functionstringToDigit :: String -> Maybe Char
which simultaneously filtered out the digits and standardised them asChar
s.I went a bit excessively Haskell with it, but I had my fun!